Arduino Read From Serial Monitor
Analog Read Serial
This example shows you how to read analog input from the physical world using a potentiometer. A potentiometer is a simple mechanical device that provides a varying amount of resistance when its shaft is turned. By passing voltage through a potentiometer and into an analog input on your board, it is possible to measure the amount of resistance produced by a potentiometer (or pot for short) as an analog value. In this example you will monitor the state of your potentiometer after establishing serial communication between your Arduino or Genuino and your computer running the Arduino Software (IDE).
Serial.println(sensorValue) Now, when you open your Serial Monitor in the Arduino Software (IDE) (by clicking the icon that looks like a lens, on the right, in the green top bar or using the keyboard shortcut Ctrl+Shift+M), you should see a steady stream of numbers ranging from 0-1023, correlating to the position of the pot. Arduino Function Serial.read and Serial.readString: Serial monitor of Arduino is a very useful feature.Serial monitor is used to see receive data, send data,print data and so on.Serial monitor is connected to the Arduino through serial communication. This serial communication occurs using RX. Convert serial.read into a useable string using Arduino? Ask Question Asked 8 years. Here is an example of it being used to read commands from the serial monitor. Convert buffer array into a useable string using Arduino?( for connecting processing) 1. I am very new to Arduino Programming. I am trying to identify the input string from serial monitor and printing the output to the console accordingly Code is: void setup Serial.begi. Jan 17, 2018 Sending numeric values from Arduinos' Serial Monitor to the Arduino board can be tricky. In this video I explain the problem, and demonstrate some basic ways of solving it. To read more about the.
Mar 19, 2015 A string is a series of characters. To be able to read a string from the serial port in the Arduino, we will need to know when the string ends. One way to do this is to insert a newline character at the end of the string. A newline character is a non-printable ASCII character that is called 'line feed' in the ASCII control code table. Jan 08, 2018 Hi forum, we are trying to make a school project with the arduino mega 2560 we want to control a servo from serial.monitor.we want to give a angle(a.
Hardware Required
- Arduino or Genuino Board
- 10k ohm Potentiometer
Circuit
Connect the three wires from the potentiometer to your board. The first goes from one of the outer pins of the potentiometerto ground . The second goes from the other outer pin of the potentiometer to 5 volts. The third goes from the middle pin of the potentiometer to the analog pin A0.
click the image to enlarge
image developed using Fritzing. For more circuit examples, see the Fritzing project page
By turning the shaft of the potentiometer, you change the amount of resistance on either side of the wiper, which is connected to the center pin of the potentiometer. This changes the voltage at the center pin. When the resistance between the center and the side connected to 5 volts is close to zero (and the resistance on the other side is close to 10k ohm), the voltage at the center pin nears 5 volts. When the resistances are reversed, the voltage at the center pin nears 0 volts, or ground. This voltage is the analog voltage that you're reading as an input.
The Arduino and Genuino boards have a circuit inside called an analog-to-digital converter or ADC that reads this changing voltage and converts it to a number between 0 and 1023. When the shaft is turned all the way in one direction, there are 0 volts going to the pin, and the input value is 0. When the shaft is turned all the way in the opposite direction, there are 5 volts going to the pin and the input value is 1023. In between, analogRead() returns a number between 0 and 1023 that is proportional to the amount of voltage being applied to the pin.
Schematic
click the image to enlarge
Code
In the sketch below, the only thing that you do in the setup function is to begin serial communications, at 9600 bits of data per second, between your board and your computer with the command:
Serial.begin(9600);
Next, in the main loop of your code, you need to establish a variable to store the resistance value (which will be between 0 and 1023, perfect for an int
datatype) coming in from your potentiometer:
int sensorValue = analogRead(A0);
Finally, you need to print this information to your serial monitor window. You can do this with the command Serial.println() in your last line of code:
Serial.println(sensorValue)
Now, when you open your Serial Monitor in the Arduino Software (IDE) (by clicking the icon that looks like a lens, on the right, in the green top bar or using the keyboard shortcut Ctrl+Shift+M), you should see a steady stream of numbers ranging from 0-1023, correlating to the position of the pot. As you turn your potentiometer, these numbers will respond almost instantly.
See Also:
- setup()
- loop()
- analogRead()
- serial
- BareMinimum - The bare minimum of code needed to start an Arduino sketch.
- Blink - Turn an LED on and off.
- DigitalReadSerial - Read a switch, print the state out to the Arduino Serial Monitor.
- Fade - Demonstrates the use of analog output to fade an LED.
Last revision 2015/07/28 by SM
I'm using two Arduinos to sent plain text strings to each other using newsoftserial and an RF transceiver.
Each string is perhaps 20-30 characters in length. How do I convert Serial.read()
into a string so I can do if x 'testing statements'
, etc.?
15 Answers
Peter MortensenYou can use Serial.readString()
and Serial.readStringUntil()
to parse strings from Serial on the Arduino.
You can also use Serial.parseInt()
to read integer values from serial.
The value to send over serial would be my stringn5
and the result would be str = 'my string'
and x = 5
I was asking the same question myself and after some research I found something like that.
It works like a charm for me. I use it to remote control my Arduino.
The best and most intuitive way is to use serialEvent() callback Arduino defines along with loop() and setup().
I've built a small library a while back that handles message reception, but never had time to opensource it.This library receives n terminated lines that represent a command and arbitrary payload, space-separated.You can tweak it to use your own protocol easily.
First of all, a library, SerialReciever.h:
To use it, in your project do this:
To use the received commands:
If you want to read messages from the serial port and you need to deal with every single message separately I suggest separating messages into parts using a separator like this:
This way you will get a single message every time you use the function.
Here is a more robust implementation that handles abnormal input and race conditions.
- It detects unusually long input values and safely discards them. For example, if the source had an error and generated input without the expected terminator; or was malicious.
- It ensures the string value is always null terminated (even when buffer size is completely filled).
- It waits until the complete value is captured. For example, transmission delays could cause Serial.available() to return zero before the rest of the value finishes arriving.
- Does not skip values when multiple values arrive quicker than they can be processed (subject to the limitations of the serial input buffer).
- Can handle values that are a prefix of another value (e.g. 'abc' and 'abcd' can both be read in).
It deliberately uses character arrays instead of the String
type, to be more efficient and to avoid memory problems. It also avoids using the readStringUntil()
function, to not timeout before the input arrives.
The original question did not say how the variable length strings are defined, but I'll assume they are terminated by a single newline character - which turns this into a line reading problem.
Here is an example of it being used to read commands from the serial monitor:
HoylenHoylenIf you're using concatenate method then don't forget to trim the string if you're working with if else method.
SandyUse string append operator on the serial.read(). It works better than string.concat()
After you are done saving the stream in a string(mystring, in this case), use SubString functions to extract what you are looking for.
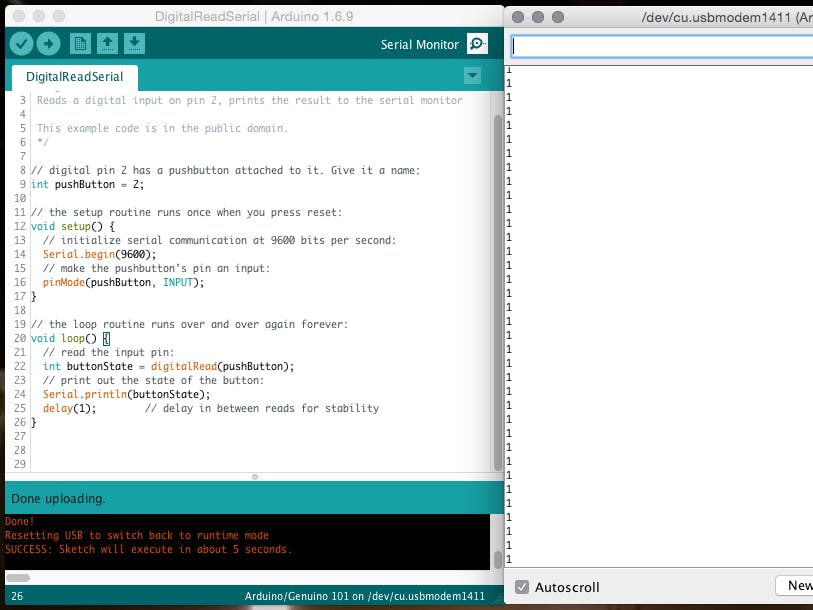
Credit for this goes to magma. Great answer, but here it is using c++ style strings instead of c style strings. Some users may find that easier.
Many great answers, here is my 2 cents with exact functionality as requested in the question.
Plus it should be a bit easier to read and debug.
Code is tested up to 128 chars of input.
Tested on Arduino uno r3 (Arduino IDE 1.6.8)
Functionality:
Correct prices and promotions are validated at the time your order is placed. Hp 3050 deskjet drivers. These terms apply only to products sold by HP.com; reseller offers may vary. Despite our best efforts, a small number of items may contain pricing, typography, or photography errors.
- Turns Arduino onboard led (pin 13) on or off using serial command input.
Commands:
- LED.ON
- LED.OFF
Note: Remember to change baud rate based on your board speed.
ZunairZunair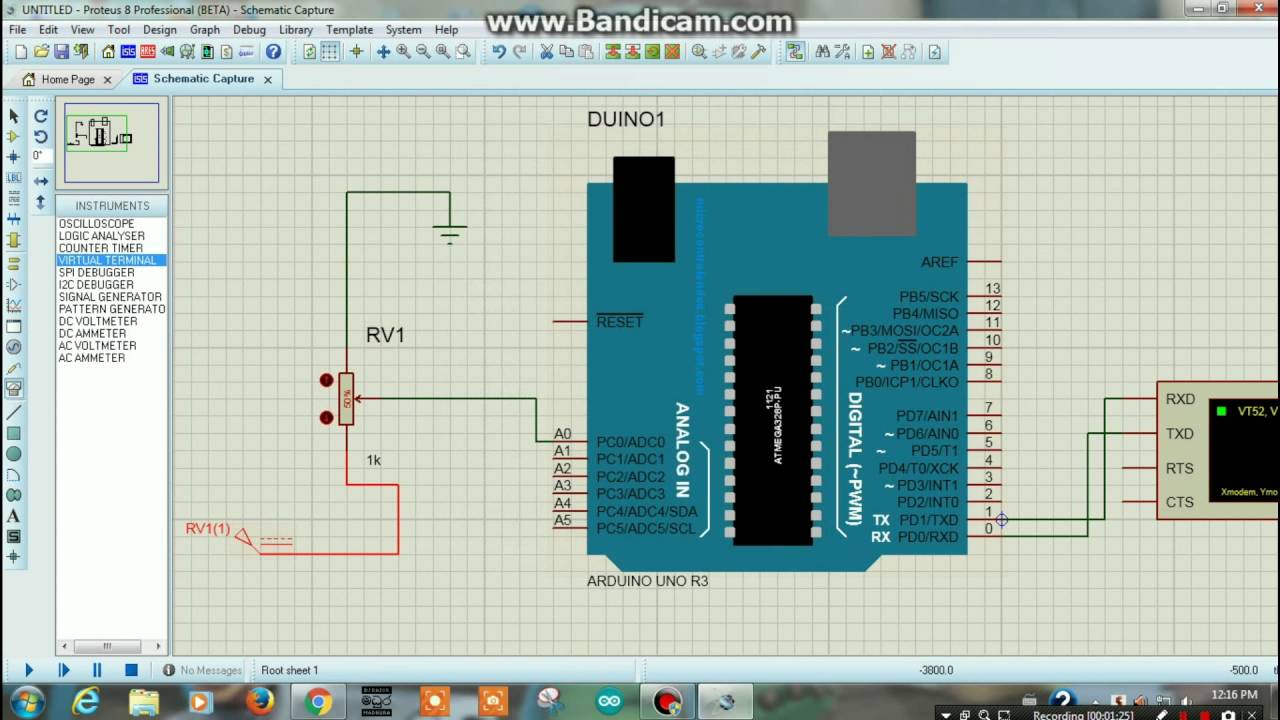
Arduino Get Input From Serial Monitor
Arduino Read Text From Serial Monitor
protected by Community♦Jan 22 '17 at 19:13
Thank you for your interest in this question. Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?